목록[ javascript ]/react (59)
IT_susu
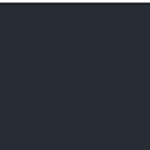
Picking a Key 키 선택하기 When we render a list, React stores some information about each rendered list item. When we update a list, React needs to determine what has changed. We could have added, removed, re-arranged, or updated the list’s items. 리스트를 렌더할 때, 리액트는 리스트 아이템을 렌더링한 정보들을 저장한다. 리스트를 업데이트 할 때, 리액트는 무엇이 바뀌었는지 결정할 필요가 잇다. 우리는 리스트의 항목들을 추가하거나 삭제하거나 재배열하거나 업데이트 할 수 있다. Imagine transitioning fro..
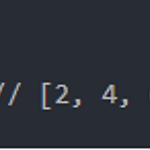
Showing the Past Moves 과거 행적 보기 Since we are recording the tic-tac-toe game’s history, we can now display it to the player as a list of past moves. 우리가 틱택토 게임의 기록을 기록했기 때문에, 우리는 보여줄 수 있다. 선수의 과거 행적을 리스트로. We learned earlier that React elements are first-class JavaScript objects; we can pass them around in our applications. To render multiple items in React, we can use an array of React elements...
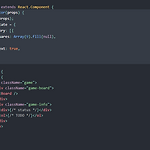
Lifting State Up, Again 다시 state를 올리자. We’ll want the top-level Game component to display a list of past moves. It will need access to the history to do that, so we will place the history state in the top-level Game component. 우리는 상위 레벨 game 컴포넌트를 원한다. 과거 동작 리스트를 보여주기 위해서. 그렇게 하기 위해서는 기록에 접근할 필요가 있다. 그래서 우리는 상위레벨인 game 컴포넌트에 history state를 놓을 것이다. Placing the history state into the Game componen..
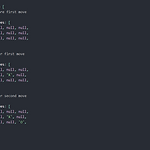
As a final exercise, let’s make it possible to “go back in time” to the previous moves in the game. 마지막 연습으로, 게임의 이전 동작에 대해 시간을 되돌리는 것을 해보자. Storing a History of Moves 동작 기록 저장 If we mutated the squares array, implementing time travel would be very difficult. 만약 squares 배열을 바꾼다면, 시간여행을 하는 것은 매우 어려울 것이다. However, we used slice() to create a new copy of the squares array after every move, and trea..
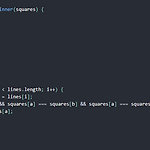
Declaring a Winner 승자 선언 Now that we show which player’s turn is next, we should also show when the game is won and there are no more turns to make. Copy this helper function and paste it at the end of the file: 이제 다음 차례 선수를 보여주었다면, 우리는 게임에서 이겼을 때 더이상 턴이 돌아가지 않도록 해야한다. helper 함수를 복사해서 파일의 끝부분에 붙여라. Given an array of 9 squares, this function will check for a winner and return 'X', 'O', or null as..
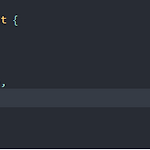
Taking Turns 턴 가져오기 We now need to fix an obvious defect in our tic-tac-toe game: the “O”s cannot be marked on the board. 틱택토 게임에서 명백한 결함을 고칠 필요가 있다.: O가 board에서 만들어지지 않는다. We’ll set the first move to be “X” by default. We can set this default by modifying the initial state in our Board constructor: 첫번째 이동은 기본적으로 X로 설정된다. 이 기본 설정을 board 생성자함수에서 초기 state를 수정함으로써 설정할 수 있다. Each time a player moves..
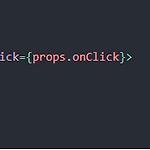
Function Components 함수 컴포넌트들 We’ll now change the Square to be a function component. 우린 이제 square를 함수형 컴포넌트로 바꿀 수 있다. In React, function components are a simpler way to write components that only contain a render method and don’t have their own state. Instead of defining a class which extends React.Component, we can write a function that takes props as input and returns what should be rendered. ..
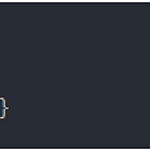
Why Immutability Is Important 불변의 중요성 In the previous code example, we suggested that you use the .slice() operator to create a copy of the squares array to modify instead of modifying the existing array. We’ll now discuss immutability and why immutability is important to learn. 이전 코드 예제에서, 우리는 slice() 명령어를 사용해서 존재하는 배열을 수정하는 것 대신 수정한 squares 배역을 복사해야 한다고 주장했었다. 이제 불변성과 불변의 중요성에 대해 토론해보자. There ..
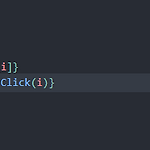
We will now use the prop passing mechanism again. We will modify the Board to instruct each individual Square about its current value ('X', 'O', or null). We have already defined the squares array in the Board’s constructor, and we will modify the Board’s renderSquaremethod to read from it: renderSquare(i) { return ; } 우리는 전달하는 메커니즘인 prop을 한번 더 사용할 것이다. 우리는 Board를 수정할 것이다. 각각의 square에게 현재 value값..
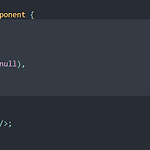
Completing the Game 게임 완성하기 We now have the basic building blocks for our tic-tac-toe game. To have a complete game, we now need to alternate placing “X”s and “O”s on the board, and we need a way to determine a winner. 우리는 이제 틱택토 게임의 토대를 쌓았다. 게임을 완성하기 위해서, 우리는 X와 O를 번갈아가며 보드에 놓는 것이 필요하고, 승자를 결정하는 방법이 필요하다. Lifting State Up state를 들어 올리다 Currently, each Square component maintains the game’s state..